
ROBOTICS:
PROJECT-BASED LEARNING
PROJECT:
BLUETOOTH RC CAR
Design
The simplest solution to this is to simply take an existing remote control car and replace the existing circuits with your new one.
You would have to ensure the car is suitable based on the size and expected power source (battery) it uses.
Remove the RC circuit, but retain the motors and existing wires to make your task easier.
Then, its only a matter of configuring your Bluetooth interface to control the motors accordingly.
Note that this is a complex task that requires a significant amount of basics in circuits and programming. Exercise caution especially with the electrical equipment.
​
​
​
​
​
​
​
​
​
​
​
​
​
WARNING:
When connecting your circuits to external power source, always ensure that the voltage is appropriate for your circuit board. High voltage levels will permanently damage sensitive electronic equipment.
DO NOT USE AC POWER (WALL SOCKETS)!
What You Need

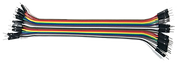
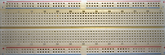
Microcontroller
(Arduino etc)
Jumper Wires
Bread
Board
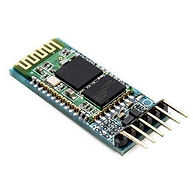
Bluetooth Module
HC-05
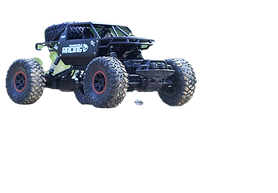
RC Car
(Old or New)
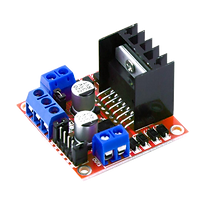
Motor Driver
L298N
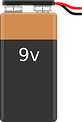
9V Battery
The Circuit

Created with Fritzing
The Code
const int motorpin1 = 5,
const int motorpin2 = 6;
const int motorpin3 = 9;
const int motorpin4 = 10;
int data = 0;
void setup(){
Serial.begin(9600);
pinMode(motorpin1, OUTPUT);
pinMode(motorpin2, OUTPUT);
pinMode(motorpin3, OUTPUT);
pinMode(motorpin4, OUTPUT);
}
​
void loop(){
if(Serial.available() > 0){
data = Serial.read();
}
if (data =='f'){
analogWrite(motorpin3, 255); //Move forward
analogWrite(motorpin4, 0);
}
else if(data=='b'){
analogWrite(motorpin3, 0); //Move backward
analogWrite(motorpin4, 255);
}
else if(data=='l'){
analogWrite(motorpin1, 255); //Move left
analogWrite(motorpin2, 0);
analogWrite(motorpin3, 255);
analogWrite(motorpin4, 0);
}
else if(data=='r'){
analogWrite(motorpin1, 0) //Move right
analogWrite(motorpin2, 255);
analogWrite(motorpin3, 255);
analogWrite(motorpin4, 0);
}
else if(data=='q'){
analogWrite(motorpin1, 255); //Reverse left
analogWrite(motorpin2, 0);
analogWrite(motorpin3, 0);
analogWrite(motorpin4, 255);
}
else if(data=='m'){
analogWrite(motorpin1, 0); //Reverse right
analogWrite(motorpin2, 255);
analogWrite(motorpin3, 0);
analogWrite(motorpin4, 255); }
else if(data=='s'){
analogWrite(motorpin1, 0); //Reverse right
analogWrite(motorpin2, 0);
analogWrite(motorpin3, 0);
analogWrite(motorpin4, 0);
}
}
The App
For ease, you may download the Android version of the bluetooth control app here
No compatible iOS available currently